The screenOrientation is the attribute of activity element. The orientation of android activity can be portrait, landscape, sensor, unspecified etc. You need to define it in the AndroidManifest.xml file. For example:
- <activity
- android:name="com.example.screenorientation.MainActivity"
- android:label="@string/app_name"
- android:screenOrientation="landscape"
- >
The common values for screenOrientation attribute are as follows:
Value | Description |
unspecified | It is the default value. In such case, system chooses the orientation. |
portrait | taller not wider |
landscape | wider not taller |
sensor | orientation is determined by the device orientation sensor. |
Android landscape mode screen orientation example
activity_main.xml
File: activity_main.xml
- <RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
- xmlns:tools="http://schemas.android.com/tools"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- android:paddingBottom="@dimen/activity_vertical_margin"
- android:paddingLeft="@dimen/activity_horizontal_margin"
- android:paddingRight="@dimen/activity_horizontal_margin"
- android:paddingTop="@dimen/activity_vertical_margin"
- tools:context=".MainActivity" >
-
- <Button
- android:id="@+id/button1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_marginLeft="66dp"
- android:layout_marginTop="73dp"
- android:text="Button"
- android:onClick="onClick"
- />
-
- <EditText
- android:id="@+id/editText1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_centerHorizontal="true"
- android:ems="10" />
-
- </RelativeLayout>
Activity class
File: MainActivity.java
- package com.example.f;
-
- import android.os.Bundle;
- import android.app.Activity;
- import android.view.Menu;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- import android.widget.EditText;
-
- public class MainActivity extends Activity{
- EditText editText1;
- Button button1;
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
-
- editText1=(EditText)findViewById(R.id.editText1);
- button1=(Button)findViewById(R.id.button1);
- }
- public void onClick(View v) {
- editText1.setText("Kundan");
- }
- }
AndroidManifest.xml
File: AndroidManifest.xml
- <?xml version="1.0" encoding="utf-8"?>
- <manifest xmlns:androclass="http://schemas.android.com/apk/res/android"
- package="com.example.screenorientation"
- android:versionCode="1"
- android:versionName="1.0" >
-
- <uses-sdk
- android:minSdkVersion="8"
- android:targetSdkVersion="16" />
-
- <application
- android:allowBackup="true"
- android:icon="@drawable/ic_launcher"
- android:label="@string/app_name"
- android:theme="@style/AppTheme" >
- <activity
- android:name="com.example.screenorientation.MainActivity"
- android:label="@string/app_name"
- android:screenOrientation="landscape"
- >
- <intent-filter>
- <action android:name="android.intent.action.MAIN" />
-
- <category android:name="android.intent.category.LAUNCHER" />
- </intent-filter>
- </activity>
- </application>
-
- </manifest>
Output:
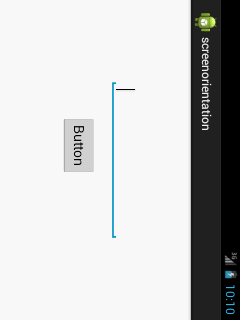
nice job on Android Online Course
ReplyDelete