Android CheckBox is a type of two state button either checked or unchecked.
There can be a lot of usage of checkboxes. For example, it can be used to know the hobby of the user, activate/deactivate the specific action etc.
Android CheckBox class is the subclass of CompoundButton class.
Android CheckBox class
The android.widget.CheckBox class provides the facility of creating the CheckBoxes.
Methods of CheckBox class
There are many inherited methods of View, TextView, and Button classes in the CheckBox class. Some of them are as follows:
Method | Description |
public boolean isChecked() | Returns true if it is checked otherwise false. |
public void setChecked(boolean status) | Changes the state of the CheckBox. |
Android CheckBox Example
activity_main.xml
Drag the three checkboxes and one button for the layout. Now the activity_main.xml file will look like this:
File: activity_main.xml
- <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
- xmlns:tools="http://schemas.android.com/tools"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- tools:context=".MainActivity" >
-
- <CheckBox
- android:id="@+id/checkBox1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignParentLeft="true"
- android:layout_alignParentTop="true"
- android:text="Pizza" />
-
- <CheckBox
- android:id="@+id/checkBox2"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignParentTop="true"
- android:layout_toRightOf="@+id/checkBox1"
- android:text="Coffe" />
-
- <CheckBox
- android:id="@+id/checkBox3"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignParentTop="true"
- android:layout_toRightOf="@+id/checkBox2"
- android:text="Burger" />
-
- <Button
- android:id="@+id/button1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_below="@+id/checkBox2"
- android:layout_marginTop="32dp"
- android:layout_toLeftOf="@+id/checkBox3"
- android:text="Order" />
-
- </RelativeLayout>
Activity class
Let's write the code to check which toggle button is ON/OFF.
File: MainActivity.java
- package com.example.checkbox;
-
- import android.os.Bundle;
- import android.app.Activity;
- import android.view.Menu;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.*;
-
- public class MainActivity extends Activity {
- CheckBox pizza,coffe,burger;
- Button buttonOrder;
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
- addListenerOnButtonClick();
- }
- public void addListenerOnButtonClick(){
-
- pizza=(CheckBox)findViewById(R.id.checkBox1);
- coffe=(CheckBox)findViewById(R.id.checkBox2);
- burger=(CheckBox)findViewById(R.id.checkBox3);
- buttonOrder=(Button)findViewById(R.id.button1);
-
-
- buttonOrder.setOnClickListener(new OnClickListener(){
-
- @Override
- public void onClick(View view) {
- int totalamount=0;
- StringBuilder result=new StringBuilder();
- result.append("Selected Items:");
- if(pizza.isChecked()){
- result.append("\nPizza 100Rs");
- totalamount+=100;
- }
- if(coffe.isChecked()){
- result.append("\nCoffe 50Rs");
- totalamount+=50;
- }
- if(burger.isChecked()){
- result.append("\nBurger 120Rs");
- totalamount+=120;
- }
- result.append("\nTotal: "+totalamount+"Rs");
-
- Toast.makeText(getApplicationContext(), result.toString(), Toast.LENGTH_LONG).show();
- }
-
- });
- }
- @Override
- public boolean onCreateOptionsMenu(Menu menu) {
-
- getMenuInflater().inflate(R.menu.activity_main, menu);
- return true;
- }
-
- }
Output:
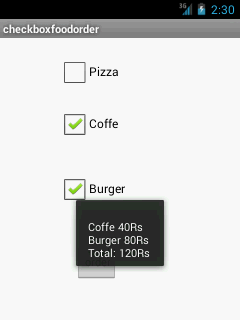
very well explained
ReplyDelete