MediaRecorder class can be used to record audio and video files.
After recording the media, we can create a sound file that can be played later.
In this example, we are going to record the audio file and storing it in the external directory in 3gp format.
activity_main.xml
Drag 2 buttons from the pallete, one to start the recording and another stop the recording. Here, we are registering the view with the listener in xml file using android:onClick.
File: activity_main.xml
- <RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
- xmlns:tools="http://schemas.android.com/tools"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- android:paddingBottom="@dimen/activity_vertical_margin"
- android:paddingLeft="@dimen/activity_horizontal_margin"
- android:paddingRight="@dimen/activity_horizontal_margin"
- android:paddingTop="@dimen/activity_vertical_margin"
- tools:context=".MainActivity" >
- <Button
- android:id="@+id/button1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignParentLeft="true"
- android:layout_alignParentTop="true"
- android:layout_marginLeft="68dp"
- android:layout_marginTop="50dp"
- android:text="Start Recording"
- android:onClick="startRecording"
- />
- <Button
- android:id="@+id/button2"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignLeft="@+id/button1"
- android:layout_below="@+id/button1"
- android:layout_marginTop="64dp"
- android:text="Stop Recording"
- android:onClick="stopRecording"
- />
- </RelativeLayout>
Activity class
File: MainActivity.java
- package com.javatpoint.mediarecorder;
- import java.io.File;
- import java.io.IOException;
- import android.app.Activity;
- import android.content.ContentResolver;
- import android.content.ContentValues;
- import android.content.Intent;
- import android.media.MediaRecorder;
- import android.net.Uri;
- import android.os.Bundle;
- import android.os.Environment;
- import android.provider.MediaStore;
- import android.util.Log;
- import android.view.View;
- import android.widget.Button;
- import android.widget.Toast;
- public class MainActivity extends Activity {
- MediaRecorder recorder;
- File audiofile = null;
- static final String TAG = "MediaRecording";
- Button startButton,stopButton;
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
- startButton = (Button) findViewById(R.id.button1);
- stopButton = (Button) findViewById(R.id.button2);
- }
- public void startRecording(View view) throws IOException {
- startButton.setEnabled(false);
- stopButton.setEnabled(true);
- //Creating file
- File dir = Environment.getExternalStorageDirectory();
- try {
- audiofile = File.createTempFile("sound", ".3gp", dir);
- } catch (IOException e) {
- Log.e(TAG, "external storage access error");
- return;
- }
- //Creating MediaRecorder and specifying audio source, output format, encoder & output format
- recorder = new MediaRecorder();
- recorder.setAudioSource(MediaRecorder.AudioSource.MIC);
- recorder.setOutputFormat(MediaRecorder.OutputFormat.THREE_GPP);
- recorder.setAudioEncoder(MediaRecorder.AudioEncoder.AMR_NB);
- recorder.setOutputFile(audiofile.getAbsolutePath());
- recorder.prepare();
- recorder.start();
- }
- public void stopRecording(View view) {
- startButton.setEnabled(true);
- stopButton.setEnabled(false);
- //stopping recorder
- recorder.stop();
- recorder.release();
- //after stopping the recorder, create the sound file and add it to media library.
- addRecordingToMediaLibrary();
- }
- protected void addRecordingToMediaLibrary() {
- //creating content values of size 4
- ContentValues values = new ContentValues(4);
- long current = System.currentTimeMillis();
- values.put(MediaStore.Audio.Media.TITLE, "audio" + audiofile.getName());
- values.put(MediaStore.Audio.Media.DATE_ADDED, (int) (current / 1000));
- values.put(MediaStore.Audio.Media.MIME_TYPE, "audio/3gpp");
- values.put(MediaStore.Audio.Media.DATA, audiofile.getAbsolutePath());
- //creating content resolver and storing it in the external content uri
- ContentResolver contentResolver = getContentResolver();
- Uri base = MediaStore.Audio.Media.EXTERNAL_CONTENT_URI;
- Uri newUri = contentResolver.insert(base, values);
- //sending broadcast message to scan the media file so that it can be available
- sendBroadcast(new Intent(Intent.ACTION_MEDIA_SCANNER_SCAN_FILE, newUri));
- Toast.makeText(this, "Added File " + newUri, Toast.LENGTH_LONG).show();
- }
- }
Output:

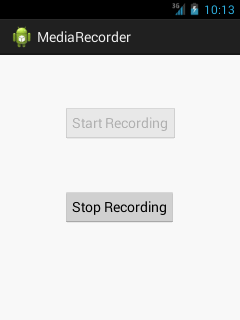
No comments:
Post a Comment